In the previous posts we have coded a few actions in Java and created them
programmatically as needed. We added them to a menu or a toolbar and could invoke them, and they worked. However, if we
search for the actions using the Find Action popup (Ctrl/Cmd+Shift+A
), we do not find them:
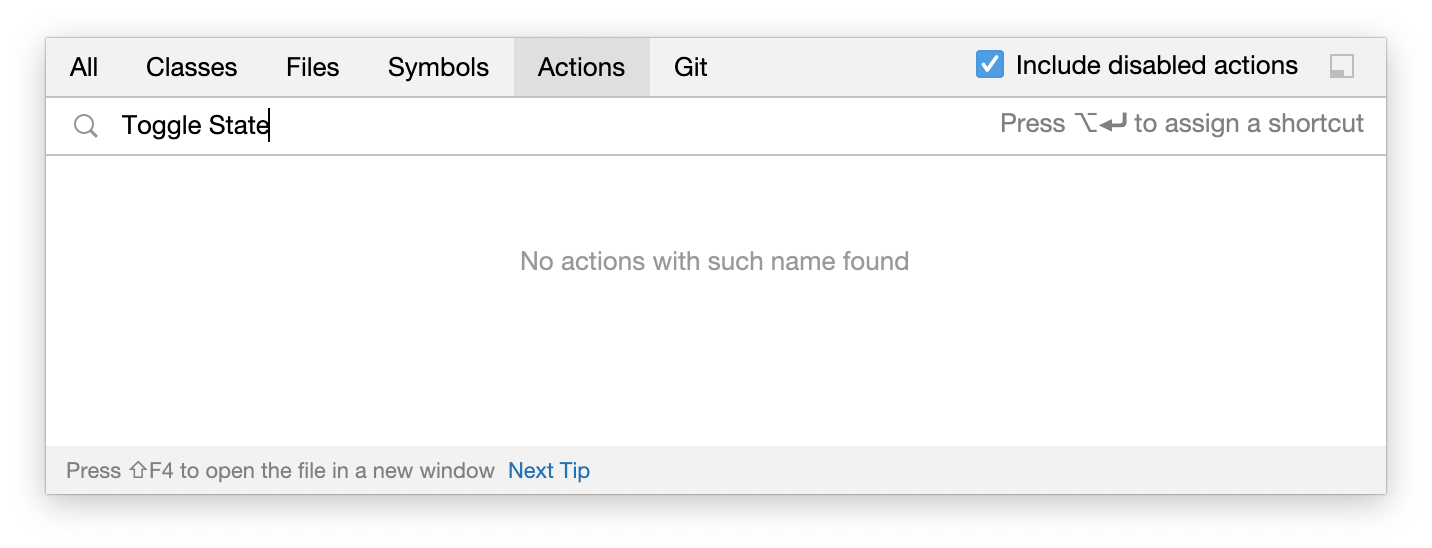
How can we make it possible to find our actions via search? We need to register them with IDEA’s ActionManager:
actionManager.registerAction("ToggleActionExample_1",
new ToggleActionExample(1));
If the module with the actions is unloaded, the actions need to be unregistered:
actionManager.unregisterAction("ToggleActionExample_1");
And instead of instantiating a ToggleActionExample
every time we need one, we should ask the ActionManager to look it
up:
// before
this.add(new ToggleActionExample(1));
// after
this.add(ActionManager.getInstance().getAction("ToggleActionExample_1"));
Where do we put the registration and unregistration code? The best place would be an application plugin because it is
only called once per application. However, putting the registration code in the plugin doesn’t work because actions and
action groups are created before our application plugin gets called, and therefore when we look up an action in our
build()
method we get null
back.
Instead, we need to resort to defensive programming and make sure the actions are registered before we query for them,
but only once. Our build()
method becomes:
ToggleActionExample.ensureActionsRegistered();
for (int i = 1; i <= 3; i++) {
this.add(ActionManager.getInstance().getAction("ToggleActionExample_" + i);
}
And here is the ensureActionsRegistered()
methods that registers the actions defensively:
public static void ensureActionsRegistered() {
ActionManager actionManager = ActionManager.getInstance();
for (int i = 1; i <= 3; i++) {
string id = "ToggleActionExample_" + i;
if (actionManager.getAction(id) == null) {
actionManager.registerAction(id, new ToggleActionExample(i));
}
}
}
The application plugin is still useful for unregistering actions. Here is what it looks like:
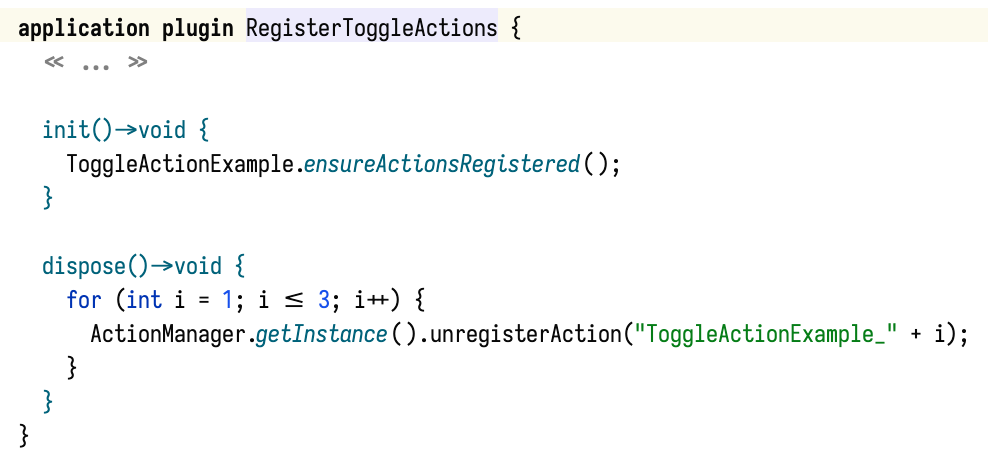
With these changes our actions now appear in the Find Action lookup:
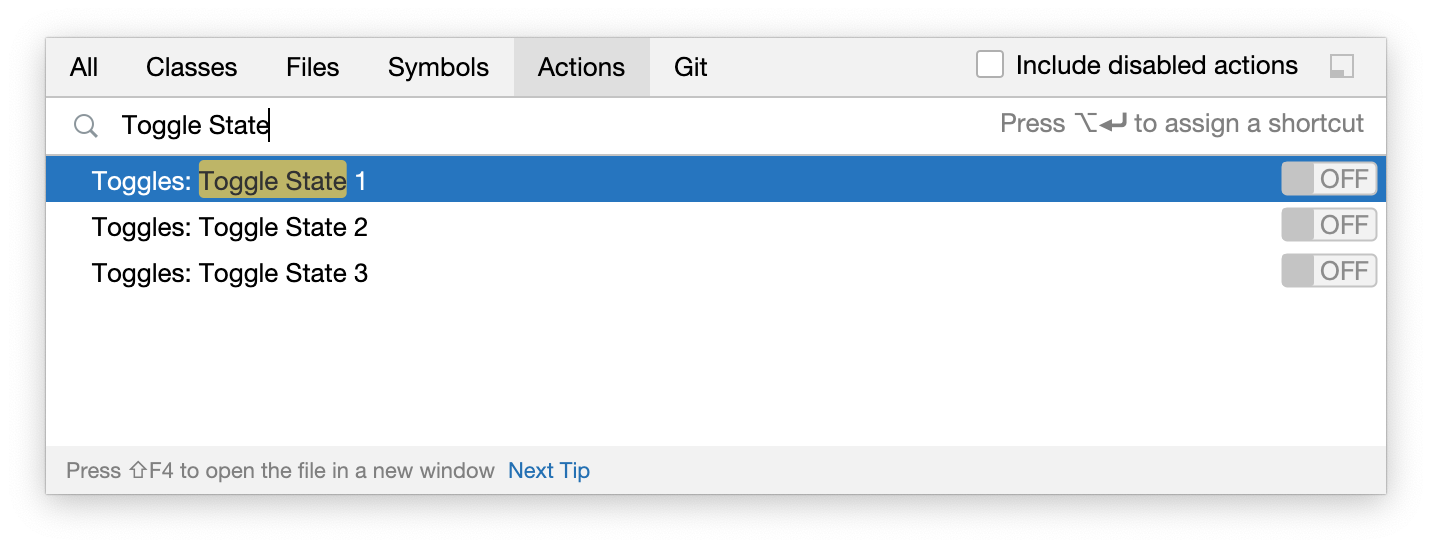
And they are also visible in the “Menus and Toolbars” settings:
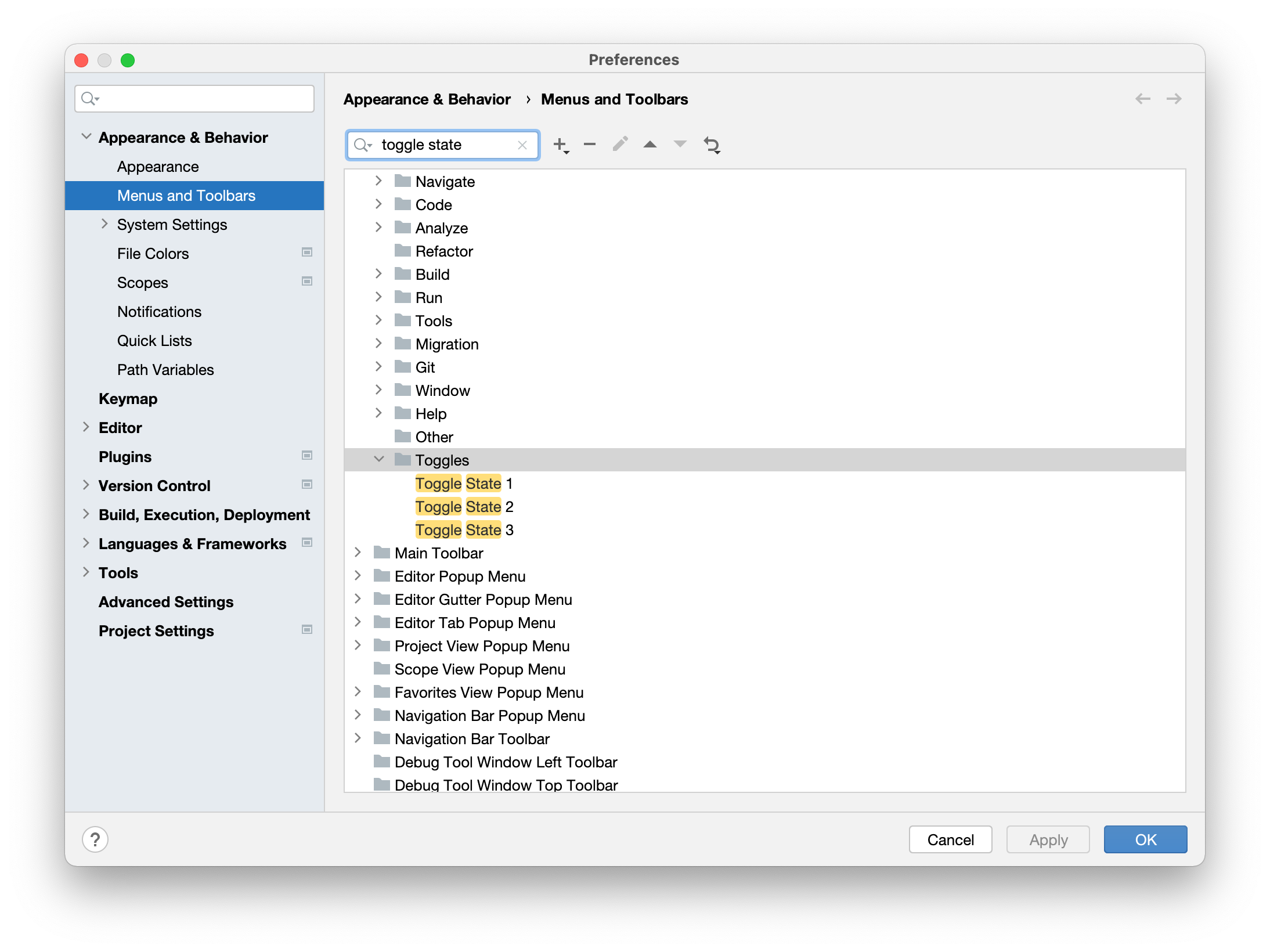
As usual, find the full code sample on GitHub.