Have you tried to add a tree view to your tool window in MPS? The default Swing trees look a bit untidy in MPS but can be made to look much nicer with a few very simple code changes.
The default, ugly tree
We start with a simple tree using the default Swing widgets, JTree
and JScrollPane
, in a tool window. This is the
code:
DefaultMutableTreeNode root = new DefaultMutableTreeNode("Root");
DefaultMutableTreeNode first = new DefaultMutableTreeNode("First");
first.add(new DefaultMutableTreeNode("1.A"));
root.add(first);
root.add(new DefaultMutableTreeNode("Second"));
root.add(new DefaultMutableTreeNode("Third"));
JTree tree = new JTree(root);
return new JScrollPane(tree);
and here is the full tool definition, if you want to follow along:
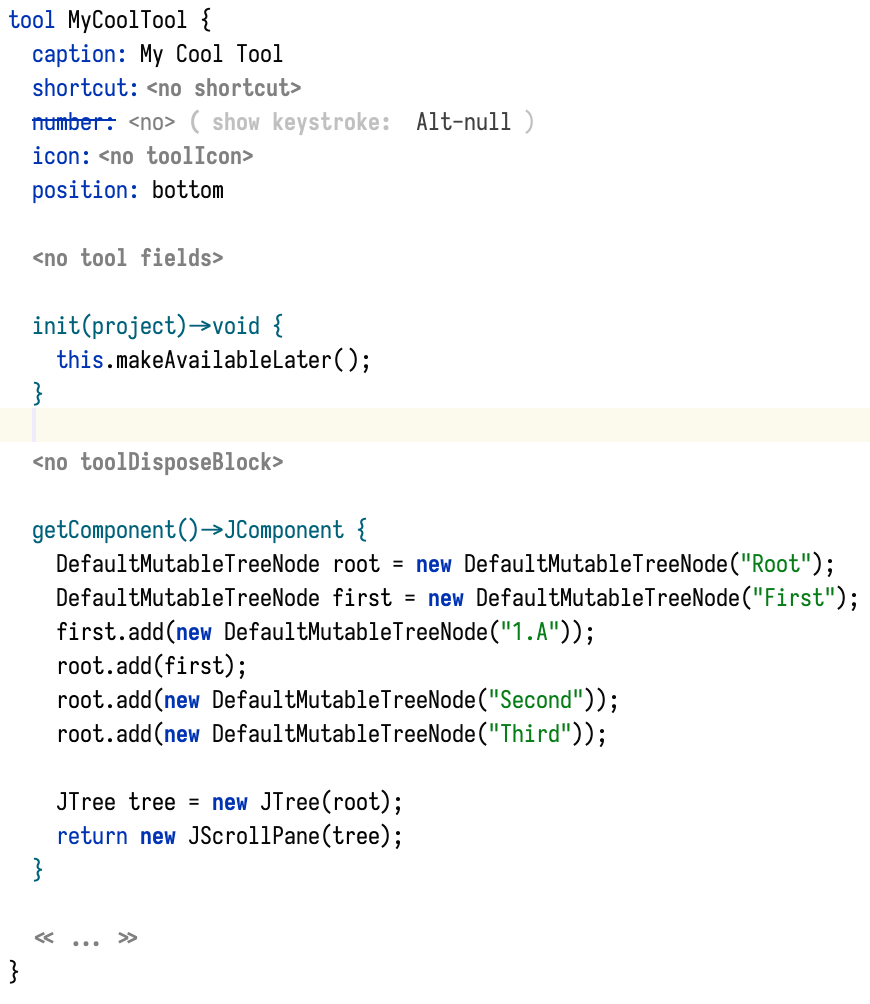
This is what the tool window it creates looks like:
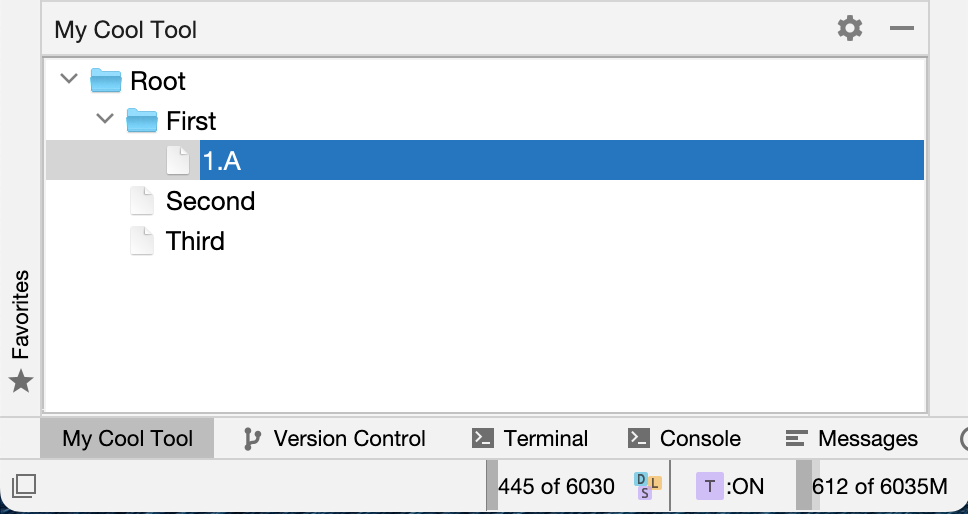
Note the thick border, the out-of-place folder icons, and the incorrectly rendered selection.
Fixing the selection
The first change we make is to switch from JTree
to com.intellij.ui.treeStructure.Tree
that is supplied by
JetBrains:
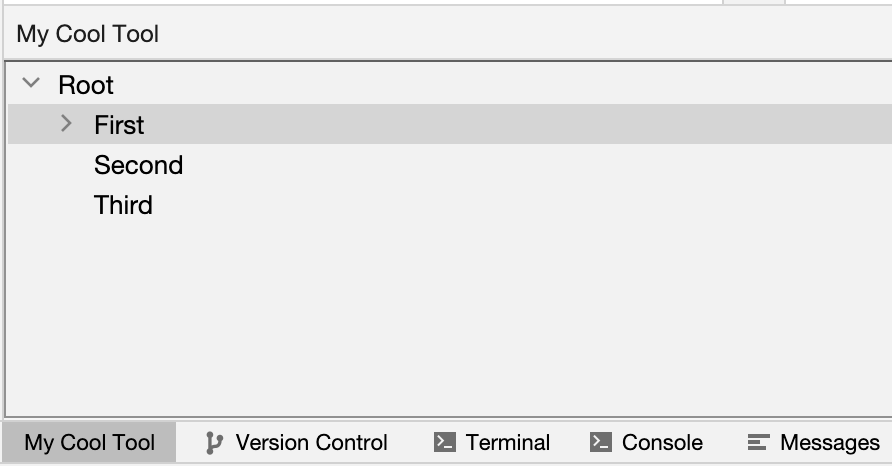
Looks like a change for the worse since the background is incorrect and the folder icons are gone completely, but at least the selection problem is gone.
Fixing the background
Switching from JScrollPane
to com.intellij.ui.components.JBScrollPane
fixes the background:
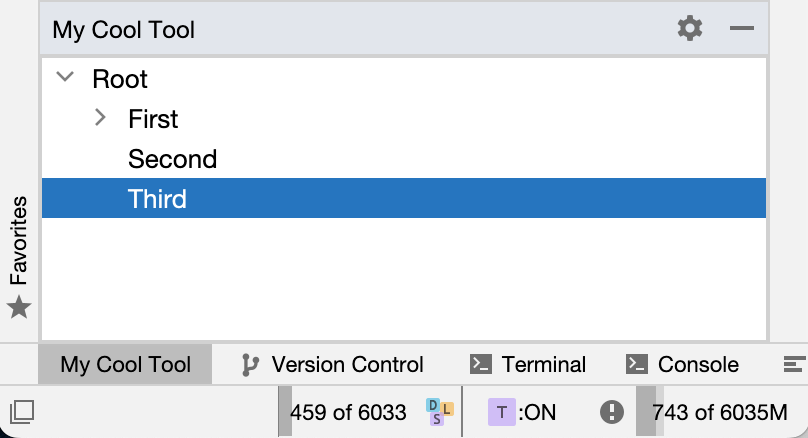
Fixing the icons
Using jetbrains.mps.ide.ui.tree.MPSTree
means writing more code but MPSTreeNodes have additional features available,
such as being able to render additional text and special support for rendering MPS nodes which comes handy. (You should
also be able to set the text color of each node individually but it didn’t work in my testing.)
Here is the modified code:
MPSTree tree = new MPSTree() {
@Override
protected MPSTreeNode rebuild() {
final MPSTreeNode root = new MPSTreeNode("Root");
root.setNodeIdentifier("Root");
MPSTreeNode first = new MPSTreeNode("First");
first.setNodeIdentifier("First");
MPSTreeNode oneA = new MPSTreeNode("1.A");
oneA.setNodeIdentifier("1.A");
oneA.setAdditionalText("extra text");
first.add(oneA);
root.add(first);
MPSTreeNode second = new MPSTreeNode("Second");
second.setNodeIdentifier("Second");
root.add(second);
MPSTreeNode third = new MPSTreeNode("Third");
third.setNodeIdentifier("Third");
root.add(third);
return root;
}
};
tree.rebuildNow();
return new JBScrollPane(tree);
The only remaining problem for us nitpickers is the double border around the tree.
Fixing the double border
To fix the double border we use com.intellij.openapi.ui.SimpleToolWindowPanel
which is a special panel for tool
windows that can render its content without an extra border. It can also render a tool bar above or to the left of the
main content widget.
We modify the code as follows:
JBScrollPane scrollPane = new JBScrollPane(tree);
SimpleToolWindowPanel stwp = new SimpleToolWindowPanel(false, true);
stwp.setContent(scrollPane);
return stwp;
And here is the final result:
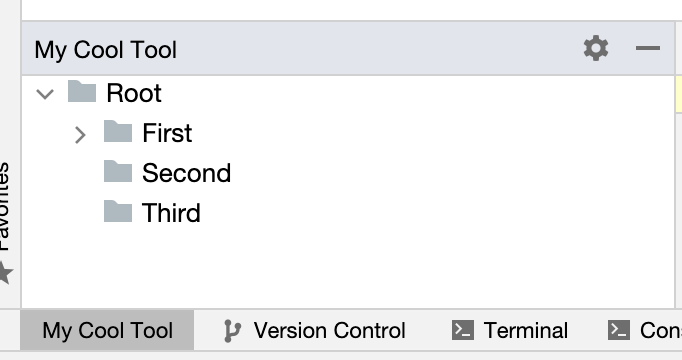
No more double borders and no more ugly trees!