You want to show an image in the editor or in a tool window. Where do you put the image and how do you access it at run time?
The traditional Java answer for this is to store the image as a resource – a non-code file stored together with .class files in a JAR. However, MPS solutions or languages don’t offer any special support or conventions for resources. What can you do?
Solution 1: a JAR with resources
The simplest way to use resources from a MPS project is to put them in a separate JAR and attach that JAR as a library to a solution or a language. For an example of this, check out the robot_Kaja MPS sample:
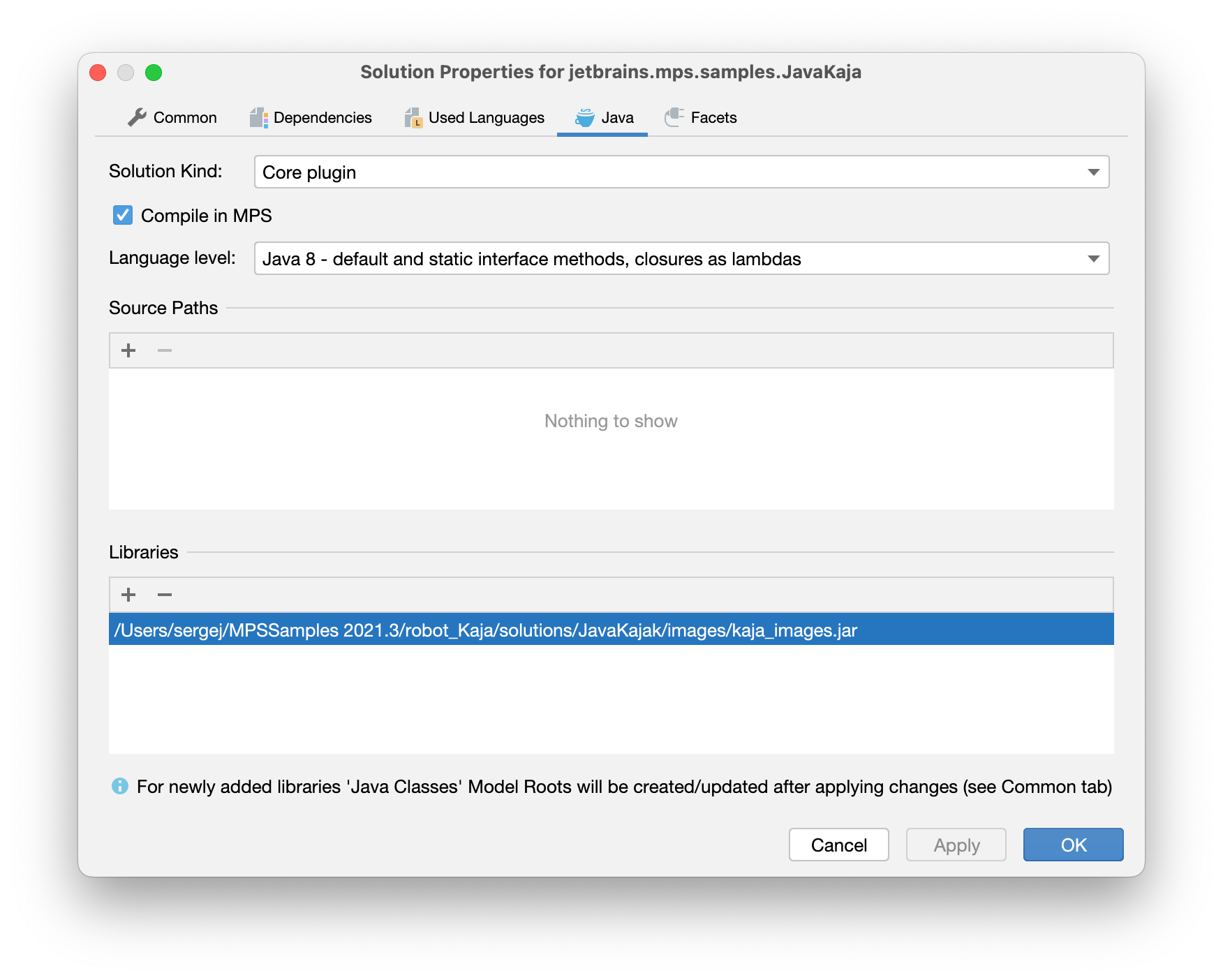
(You don’t need to add a model root for the JAR if you are only going to use it to load resources.)
At run time the library is loaded by the module class loader and you can use that class loader to access the resources
via getResourceAsStream()
or getResource()
.
Solution 2: more convenience but more code
Packaging the resources into a JAR requires extra work. If you want to save on that work, you can write a utility function to load the resource from the file system during development and from the class loader once the module is deployed (packaged).
Here is some code to get you started, assuming you put resources under the resources
subdirectory of the module
directory:
public static InputStream getResourceAsStream(SModule module,
String relativePath)
throws IOException {
if (module.isPackaged()) {
ClassLoader classLoader = ((ReloadableModule) module).getClassLoader0();
return classLoader.getResourceAsStream(relativePath);
} else {
IFile moduleDir = ((ReloadableModuleBase) module).getModuleSourceDir();
IFile resourcesDir = moduleDir.findChild("resources");
IFile resourceFile = IFileUtil.getDescendant(resourcesDir, relativePath);
return resourceFile.openInputStream();
}
}
You also need to ensure that the resources are packaged into the JAR. This is specified in the build solution:
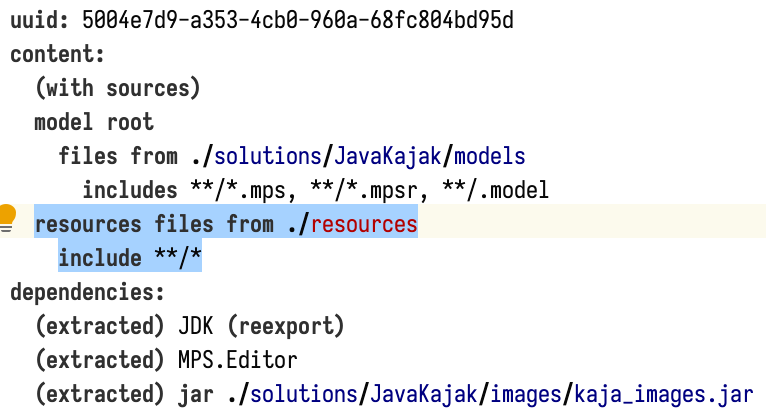